Either
Either[edit]
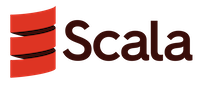
The Either type in Scala is a powerful construct that allows developers to handle situations where a computation may result in one of two possible outcomes. It represents a value that can take one of two alternative types, usually referred to as left and right. This construct can be used to elegantly handle error cases, manage branching logic, or model other scenarios where a value may not have a unique outcome.
Usage[edit]
To define an either value in Scala, the Either trait is used. The Either trait is an abstract class with two subclasses, Left and Right, representing the left and right alternatives, respectively. The left type can be any type, but the right type must be specified.
Here is an example of creating and using an Either value:
```scala val result: Either[String, Int] = if (condition) Right(42) else Left("Condition failed") result match {
case Right(value) => println(s"Success: $value") case Left(error) => println(s"Error: $error")
} ```
In this example, we create an Either value that can hold either a String (in case of an error) or an Int (in case of success). Depending on the condition, we either create a Right with the value 42 or a Left with the error message "Condition failed". We then pattern match on the result to handle the two possible outcomes.
Handling Errors[edit]
One of the most common use cases for the Either type is error handling. By convention, the Left type is often used to represent the error type, while the Right type represents the successful outcome.
Here is an example of error handling using Either:
```scala def divide(dividend: Int, divisor: Int): Either[String, Int] = {
if (divisor == 0) Left("Division by zero") else Right(dividend / divisor)
}
val result: Either[String, Int] = divide(10, 0) result match {
case Right(value) => println(s"Result: $value") case Left(error) => println(s"Error: $error")
} ```
In this example, the divide function takes two integers and returns either a Left with the error message "Division by zero" if the divisor is zero, or a Right with the result of the division. The result is then pattern matched to handle the two cases.
Chaining Computations[edit]
The Either type can also be used to chain computations together, where each step may result in a different type of outcome. This is particularly useful when working with sequential operations that depend on the success of previous steps.
Here is an example that demonstrates computation chaining with Either:
```scala def computeA(): Either[String, Int] = ??? def computeB(input: Int): Either[String, Int] = ??? def computeC(input: Int): Either[String, Int] = ???
val result: Either[String, Int] =
for { a <- computeA() b <- computeB(a) c <- computeC(b) } yield c
result match {
case Right(value) => println(s"Result: $value") case Left(error) => println(s"Error: $error")
} ```
In this example, computeA, computeB, and computeC are functions that may return an Either value. By using a for comprehension, we can chain these computations together. If any step in the chain returns a Left, the computation is short-circuited, and the error is propagated up. Otherwise, the final Right value is returned.
Conclusion[edit]
The Either type in Scala provides a powerful way to manage scenarios where a computation may have two alternative outcomes. By leveraging the left and right alternatives, developers can handle errors, manage branching logic, or chain together sequential operations. Understanding and utilizing the Either type can greatly enhance the expressiveness and reliability of Scala code.
For more information on related topics, you may want to check out the following articles:
- Option: Another type for representing optional values in Scala.
- Try: A type that represents the outcome of an operation that may either result in a value or throw an exception.
- Pattern matching: A powerful feature in Scala that allows for concise, yet expressive, handling of different cases and types.
- Functional programming: A paradigm that emphasizes the use of immutable data and pure functions.
- Error handling: A guide to different error handling techniques in Scala.
- Scala collections: An overview of the various collections available in the Scala standard library.
Note: Please note that the actual content of the linked articles may vary based on the Wiki content.