Monad
Monad[edit]
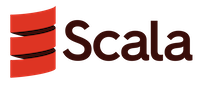
In functional programming, a monad is a design pattern and a way to structure code. It provides a standardized way to capture and sequence computations, allowing for better composition and handling of side effects. Monads originated from the mathematical field of category theory and have become an essential concept in many programming languages, including Scala.
Introduction[edit]
In Scala, a monad is an abstraction used to represent computations. It allows developers to express complex operations in a concise and composable way. Monads enable the separation of concerns by providing a mechanism to handle side effects, such as I/O or state changes, in a controlled manner.
Benefits of Monads in Scala[edit]
Monads provide several benefits when working with functional programming in Scala. Some of these benefits include:
1. Composition: Monads enable developers to compose multiple operations together in a declarative and readable manner. This makes code easier to understand and reason about.
2. Separation of Concerns: With monads, it is possible to separate pure functional code from impure code that deals with side effects. This separation improves code maintainability and testability.
3. Error Handling: Monads facilitate error handling by providing a convenient way to handle and propagate errors throughout a computation. This simplifies error handling logic and ensures the code remains robust.
4. Concurrency: Monads can also be used to model concurrent computations, allowing for better control and management of concurrency-related issues.
Common Monad Types in Scala[edit]
Scala provides several built-in monad types, each suited for different purposes. Some of the commonly used monad types in Scala include:
1. Option: The Option
monad represents values that may or may not be present, allowing developers to handle null or undefined values in a safe and concise manner.
2. Either: The Either
monad represents a value that can be either a successful result or a failure. It is commonly used to handle error conditions and propagate errors through computations.
3. Future: The Future
monad represents a value that may not be available immediately but will be available at some point in the future. It enables asynchronous and concurrent programming in Scala.
4. Try: The Try
monad captures the result of a computation that may succeed or fail. It simplifies error handling by providing a unified way to handle exceptions and recover from failures.
5. List: The List
monad allows for the composition of computations that produce multiple results. It is widely used for handling collections and iterating over elements.
Monad Laws[edit]
To be considered a valid monad, a type in Scala must satisfy three fundamental laws:
1. Left Identity: unit(x).flatMap(f)
is equivalent to f(x)
. The unit
operation lifts a value into the monadic context, and flatMap
combines successive computations.
2. Right Identity: m.flatMap(unit)
is equivalent to m
. Chaining a computation with flatMap
and then lifting it with unit
should not change the original computation.
3. Associativity: m.flatMap(f).flatMap(g)
is equivalent to m.flatMap(x => f(x).flatMap(g))
. The order in which computations are chained should not affect the final result.
These laws ensure that monads compose and behave predictably, allowing for better code organization and reasoning.
Using Monads in Scala[edit]
To work with monads in Scala, developers utilize the monadic operations provided by each monad type. These operations usually include flatMap
, map
, and getOrElse
, among others.
For example, let's consider the Option
monad. It provides a way to safely handle nullable values:
val maybeName: Option[String] = Some("John")
val maybeAge: Option[Int] = None
val message = for {
name <- maybeName
age <- maybeAge
} yield s"Hello, $name! Your age is $age."
val result = message.getOrElse("Unknown")
In the above example, the for
comprehension enables us to sequence computations that work with values wrapped in the Option
monad. If any of the values in the sequence is None
, the overall result will be None
.
Conclusion[edit]
Monads play a crucial role in functional programming with Scala, providing powerful abstractions for structuring computations and handling side effects. Understanding monads and their usage in Scala allows developers to write more concise, maintainable, and robust code.